So – you want a step by step guide to deploying web apps to azure web apps from azure devops and changing config files in the release pipelines? Look no further.
First create the new web.config files, as seen below
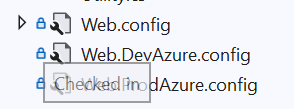
In the file properties – make sure they are set to “Content” and “Copy Always”

In the pipeline properties – make sure that xml transformation is checked, and that there is the proper value of
-ASPNETCORE_ENVIRONMENT ProdAzure
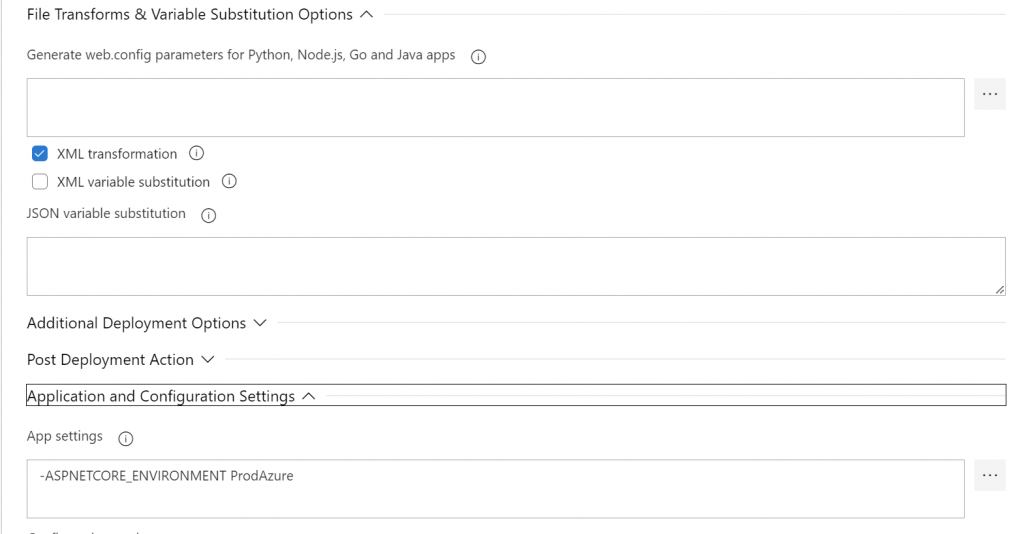
In Make sure your azure stage has the same name as the web.{name}.config file
